Put a relative clock on your Desktop with GeekTool
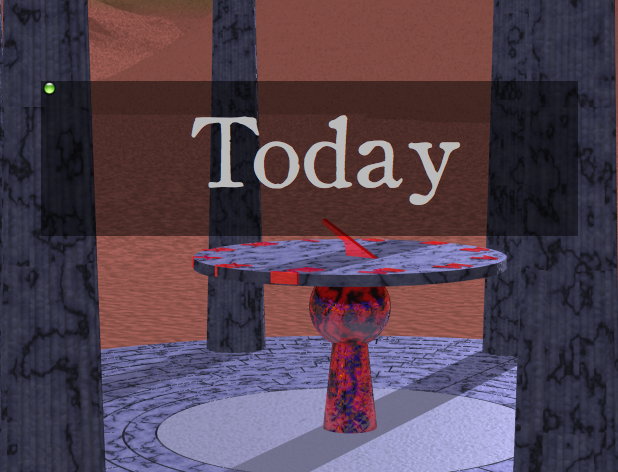
In this example, the current time is today.
Most clocks show time in a complicated display of day, month, year, hours, minutes, and seconds. But how often have you just wanted to know whether the time is today, yesterday, or last week? This Python-based GeekTool geeklet will display the relative date on your desktop.
[toggle code]
- #!/usr/bin/python
- # -*- coding: utf-8 -*-
- import optparse, datetime
- days = ('yesterday', 'today', 'tomorrow')
- parser = optparse.OptionParser('%prog')
- parser.add_option('-d', '--day', type='choice', choices=days, default='today')
- (options, slugs) = parser.parse_args()
- today = datetime.datetime.fromordinal(datetime.date.today().toordinal())
- future20 = datetime.datetime.now() + datetime.timedelta(minutes=20)
- oneDay = datetime.timedelta(days=1)
- oneWeek = datetime.timedelta(days=7)
-
if options.day == 'today':
- date = today
-
elif options.day == 'tomorrow':
- date = today + datetime.timedelta(days=1)
-
elif options.day == 'yesterday':
- date = today - datetime.timedelta(days=1)
-
else:
- date = future20
- #the future
-
if date >= today:
-
if date == future20:
- print "20 minutes into the future"
-
elif date == today:
- print "Today"
-
elif date <= today + oneDay:
- print "Tomorrow"
-
elif date <= today + oneWeek:
- print "Next", date.strftime('%A')
-
elif (date.year == today.year and date.month != today.month) or (date.year == today.year+1 and date.month <= today.month):
- print "Next", date.strftime('%B')
-
else:
- timeToFuture = date - today
- print "In", timeToFuture.days, "Days"
-
if date == future20:
- #the past
-
elif date >= today - oneDay:
- print "Yesterday"
-
elif date >= today - oneWeek:
- print "Last", date.strftime('%A')
-
elif (date.year == today.year and date.month != today.month) or (date.year == today.year-1 and date.month >= today.month):
- print "Last", date.strftime('%B')
-
else:
- timeToPast = today - date
- print timeToPast.days, "Days Ago"
If the current time is today, the script displays “today”. If it’s yesterday or tomorrow, then it displays that. If it’s within one week in either direction, it displays “next” or “last” and then the weekday name. And if it’s within the last or next twelve months, it does the same for the month name. Otherwise, it just says how many days to or since the current date.
The script uses Python’s datetime and timedelta classes to make these checks. I would have liked to use datetimes, dates, and timedeltas, but dates and datetimes are very different classes; there’s a datetime.date() method, for example, but no date.datetime(), and the datetime.today() method is really just datetime.now().
The timedelta class is used to create, basically, a time range to subtract from or add to a datetime; and when you subtract one datetime from another datetime, the result is a timedelta of the difference.
If you’d rather see how tomorrow relates to the current time, or how yesterday relates to the current time, specify “tomorrow” or “yesterday” to the --day option on the command line.
I haven’t had any need to display times, so I haven’t programmed it. If you end up needing times, however, it shouldn’t be difficult to extend the pattern I’ve started here to go down to hours or even minutes or seconds.
Keep this on your Desktop and you’ll never forget what today is!
- GeekTool
- “GeekTool is a System Preferences module for Mac OS 10.5. It lets you display on your desktop different kind of informations, provided by 3 default plugins.” The plugins let you monitor files (such as error logs), view images (such as live graphs), and display the results of command-line scripts.
More April Fool’s
- Variant Species: The Golden Servant
- “I am a golden god.” Well, a golden hero, anyway. But you’re a golden hero subject to the whims of unknown sorcerors. The Golden Servant is the perfect specialty species for the sporadic player.
- I’m stuck in a computer program; now what?
- “Help, I’ve been sucked into a computer program! What do I do?” Handy tips for how to successfully extricate yourself from the most hopeless of spaghetti code, the inner universe loop.
- Can I legally use Gary Gygax’s name for my son?
- It’s probably best to talk to a lawyer, or just avoid the issue altogether. Gaming copyright is a very complex issue, and best left to the experts or those with deep pockets. Have you considered naming him Sue?
- Secular Humanist Pantheon
- The Secular Humanist cult, while often oppressed, attracts intelligent, creative worshippers who subscribe to a rich and storied mythology. It will make a great addition to your role-playing game alongside more commonly-role-played mythologies such as Christianity, Buddhism, and Bokonism.
More GeekTool
- Bluetooth battery early warning system
- Use GeekTool, or crontab or launchd and notifications, to know when your bluetooth batteries need recharging.
- icalBuddy and eventsFrom/to
- Ali Rantakari’s icalBuddy has an error in the documentation for the “eventsFrom/to” command-line option. Rather than “tomorrow at time” use “time tomorrow”.
- Apple Mail on the Desktop with GeekTool
- Here’s a simple AppleScript to use with GeekTool to put your inbox on the Desktop.
- GeekTool, TaskPaper, and XML
- A script to convert a TaskPaper file to XML so as to filter it for specific tags and display the results on the Desktop.
- GeekTool, Perl, and ANSI color codes
- GeekTool is a great way to display the results of little scripts on your desktop. Using ANSI color codes can make those scripts even more useful. You can also change the status of the status button from “success” to “failure” depending on your script’s exit code.
- One more page with the topic GeekTool, and other related pages
More Python
- Quick-and-dirty old-school island script
- Here’s a Python-based island generator using the tables from the Judges Guild Island Book 1.
- Astounding Scripts on Monterey
- Monterey removes Python 2, which means that you’ll need to replace it if you’re still using any Python 2 scripts; there’s also a minor change with Layer Windows and GraphicConverter.
- Goodreads: What books did I read last week and last month?
- I occasionally want to look in Goodreads for what I read last month or last week, and that currently means sorting by date read and counting down to the beginning and end of the period in question. This Python script will do that search on an exported Goodreads csv file.
- Test classes and objects in python
- One of the advantages of object-oriented programming is that objects can masquerade as each other.
- Timeout class with retry in Python
- In Paramiko’s ssh client, timeouts don’t seem to work; a signal can handle this—and then can also perform a retry.
- 30 more pages with the topic Python, and other related pages